posted on 2018.2.28
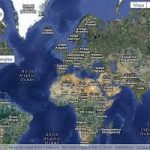
プログラムの流れ
すべてのプログラムは以下になります。大きな流れとしては次の3つです。
1.ワードプレスに取得したApiキーを登録する。
2.住所をジオコーディングして必要なデータを取得する。
3.取得したデータをもとにショートコードでマップを表示する。
次のページ以降でおのおのを簡単に説明します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 |
<?php /* Plugin Name: Bit Gmaps Short Code Plug Description: wordpressにshortcodeでグーグルマップを挿入する Version: 1.1 Author:fumiaki komori Author URI: http://bi-t-web.com/ License: ご自由 */ /** 1.ワードプレスにApiキーを登録する * */ function add_scripts() { wp_enqueue_script('google-maps', 'https://maps.googleapis.com/maps/api/js?key=your api key'); } add_action('wp_enqueue_scripts', 'add_scripts'); /** 3. ショートコードでグーグルマップを挿入する */ function bit_gmap_shortcode($atts) { //後から変更したいグーグルマップの属性値のデフォルトを登録する $atts = shortcode_atts( array( 'address' => false, 'width' => '100%', 'height' => '250px', 'zoom' => 17, ), $atts ); $address = $atts['address']; if ($address) : //キャッシュしてあるジオコードのデータの取得 $coordinates = bit_gmap_get_coordinates($address); if (!is_array($coordinates)) return; //一意IDの生成 $map_id = uniqid('b_map_'); //Google Maps JavaScript API でマップを表示する ob_start(); ?> <div class="b_map_canvas" id="<?php echo esc_attr($map_id); ?>" style="height: <?php echo esc_attr($atts['height']); ?>; width: <?php echo esc_attr($atts['width']); ?>"></div> <script type="text/javascript"> var map_<?php echo $map_id; ?>; function bit_run_map_<?php echo $map_id; ?>() { var location = new google.maps.LatLng("<?php echo $coordinates['lat']; ?>", "<?php echo $coordinates['lng']; ?>"); var map_options = { zoom: <?php echo $atts['zoom']; ?>, center: location, } map_<?php echo $map_id; ?> = new google.maps.Map(document.getElementById("<?php echo $map_id; ?>"), map_options); /* icon begin */ var markerOptions = { position: location, map: map_<?php echo $map_id; ?>, }; var marker = new google.maps.Marker(markerOptions); } bit_run_map_<?php echo $map_id; ?>(); </script> <?php return ob_get_clean(); else : return __('This Google Map cannot be loaded because the maps API does not appear to be loaded', 'bit-gmaps-short-code'); endif; } //ワードプレスにショートコードを登録 add_shortcode('bit_gmap', 'bit_gmap_shortcode'); /** 2.住所をジオコーディングする * */ function bit_gmap_get_coordinates($address, $force_refresh = false) { $address_hash = md5($address); $coordinates = get_transient($address_hash); if ($force_refresh || $coordinates === false) { //geocode用アドレスの生成 $args = array('address' => urlencode($address)); $url = add_query_arg($args, 'http://maps.googleapis.com/maps/api/geocode/json'); //geocodeデータを取得 $response = wp_remote_get($url); if (is_wp_error($response)) return; //取得したgeocodeデータからbodyの配列を抜き出す $data = wp_remote_retrieve_body($response); if (is_wp_error($data)) return; if ($response['response']['code'] == 200) { //jsonデータをphpで読めるようにデコードする $data = json_decode($data); if ($data->status === 'OK') { //必要なデータを$cache_value配列に挿入。 $coordinates = $data->results[0]->geometry->location; $cache_value['lat'] = $coordinates->lat; $cache_value['lng'] = $coordinates->lng; $cache_value['address'] = (string) $data->results[0]->formatted_address; // cache coordinates for 3 months 抜き出したデータを3ヶ月間キャッシュします。 set_transient($address_hash, $cache_value, 3600 * 24 * 30 * 3); $data = $cache_value; } else { return __('Something went wrong while retrieving your map, please ensure you have entered the short code correctly.', 'simple-google-maps-short-code'); } } else { return __('Unable to contact Google API service.', 'simple-google-maps-short-code'); } } else { $data = $coordinates; } // キャッシュデータを返す return $data; } |